Colab Notebooks
All of the methods for working with notebooks in EDSL are available in Colab.
To access these methods in Colab, please first complete the following steps to set up EDSL and connect your Google Drive:
1. Store your API key as a secret
In Google Colab, your API keys can be stored as “secrets” in lieu of storing them in a .env file as you would in other notebook types.
For example, you can store your Expected Parrot API key as follows:
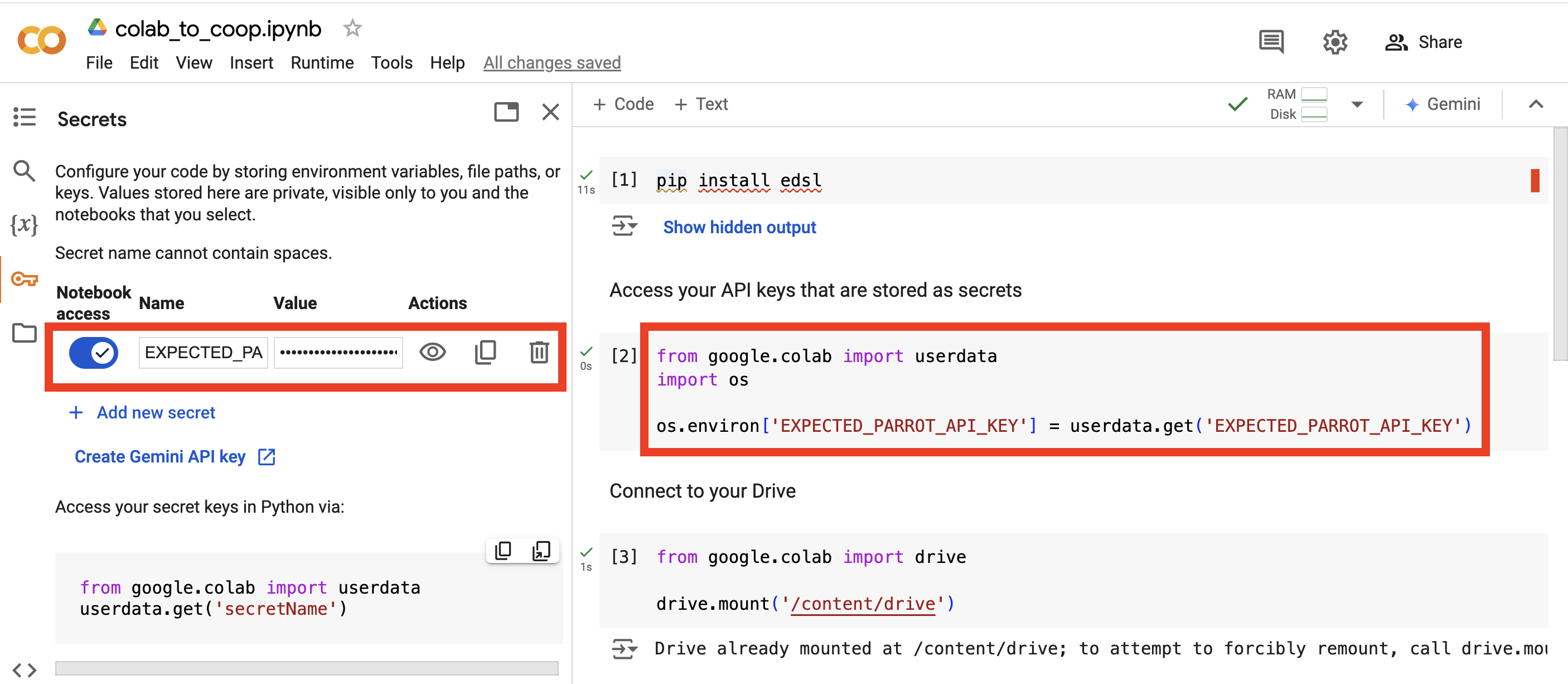
2. Install EDSL
Run the following command in a code cell to install the EDSL package:
pip install edsl
3. Access your API key
Run the following code to access your API key in your Colab notebook:
import os
from google.colab import userdata
os.environ['EXPECTED_PARROT_API_KEY'] = userdata.get('EXPECTED_PARROT_API_KEY')
4. Connect your Google Drive
To access your files in Google Drive, you need to connect your Google Drive to Colab. Run the following code to do this, and accept the permissions request in the pop-up window:
from google.colab import drive
drive.mount('/content/drive')
You will see the following message returned:
Drive already mounted at /content/drive; to attempt to forcibly remount, call drive.mount("/content/drive", force_remount=True).
5. Access your files
Run the following code to see the names of all the files in a Google Drive folder
Note that you will need to replace the path with the path to your own folder, and you may need to adjust the path to match the structure of your Google Drive (e.g., here the default Google Drive folder name “Colab Notebooks” has been changed to “ColabNotebooks” for convenience in specifying the path):
import os
print(sorted(os.listdir('/content/drive/MyDrive/ColabNotebooks/')))
Sample output:
['colab_to_coop.ipynb']
6. Post a notebook to Coop
Now that you have your notebook ready, you can post it to Coop using the Notebook object. Modify the path to your notebook file path in your Google Drive as needed:
from edsl import Notebook
notebook = Notebook(path="/content/drive/MyDrive/ColabNotebooks/colab_to_coop.ipynb")
notebook.push(description="Posting a Colab notebook to Coop")
Example output:
{'description': 'Posting a Colab notebook to Coop',
'object_type': 'notebook',
'url': 'https://www.expectedparrot.com/content/a878656a-317a-4181-a496-3c49f12e38d7',
'uuid': 'a878656a-317a-4181-a496-3c49f12e38d7',
'version': '0.1.36',
'visibility': 'unlisted'}
7. Update or edit a notebook at Coop
from edsl import Notebook
notebook = Notebook(path="/content/drive/MyDrive/ColabNotebooks/colab_to_coop.ipynb")
notebook.patch(
uuid = "a878656a-317a-4181-a496-3c49f12e38d7",
visibility = "public",
value = notebook
)
Output:
{'status': 'success'}
Example Colab code
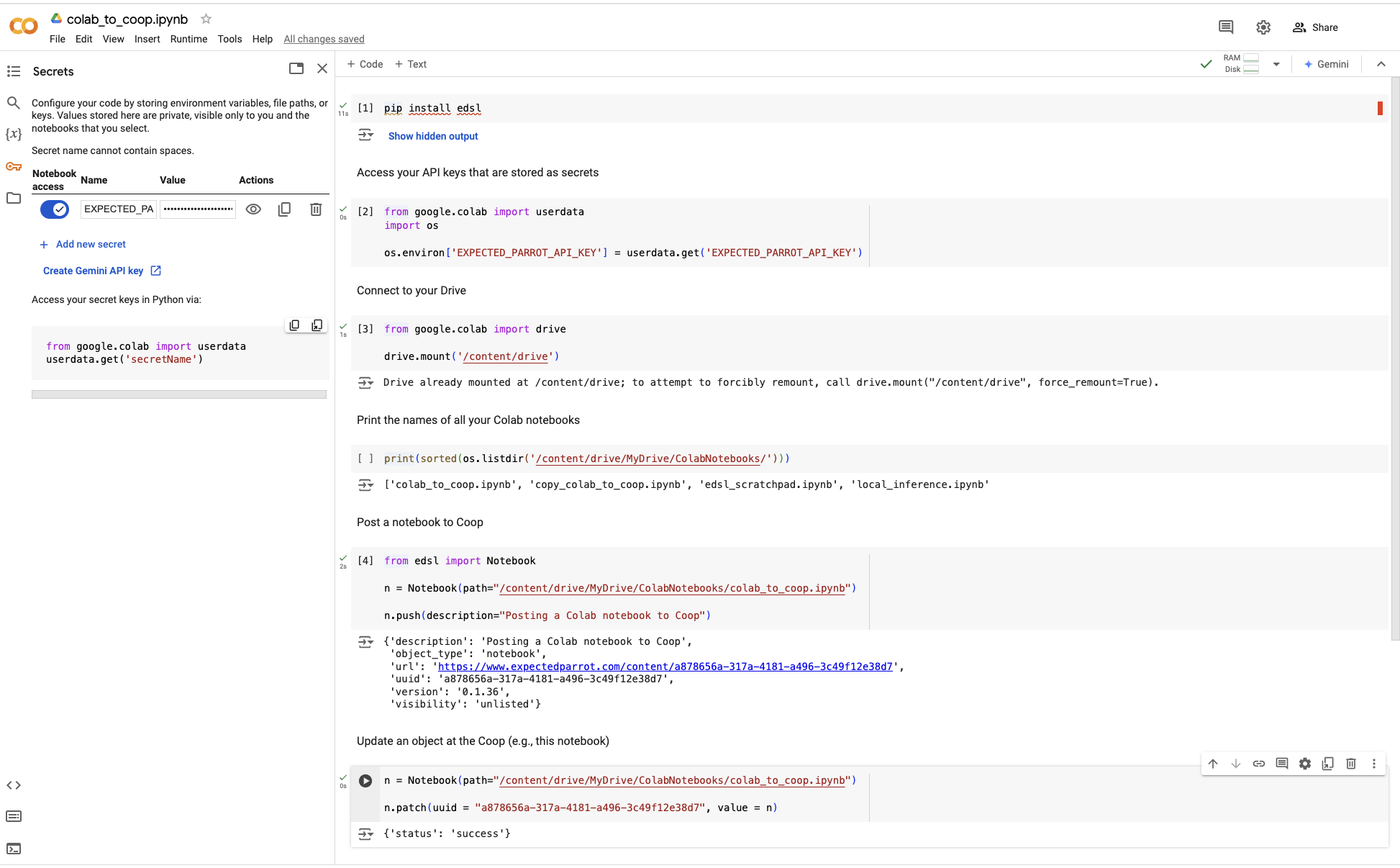